Essay COMP 220 Week 1 Lab Two Dimensional Arrays
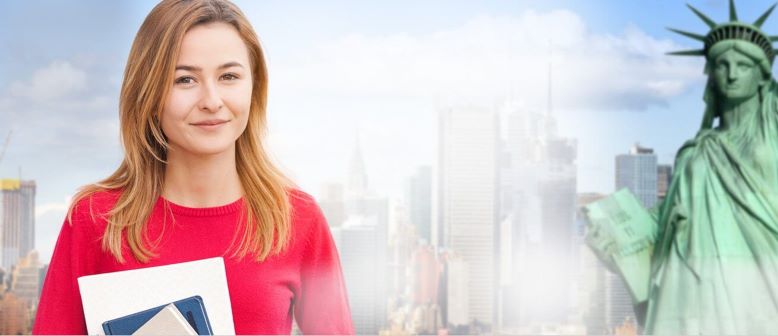
COMP 220 Week 1 Lab Two Dimensional Arrays
This lab requires you to design and implement a C++ program to simulate a game of Blackjack between two to four players. Your program must incorporate a two-dimensional array to represent the suit and the value of each card dealt to a player, keep track of which cards have been dealt to which player, and use a random-number generator to pick each card to be dealt to a player.
Deliverables
- Submit a single Notepad file containing the source code for Lab 1 to the Dropbox for Week 1. Your source code should use proper indentation and be error free. Be sure that your last name and the lab number are part of the file name: for example,
Y ourLastName_Lab1.txt.
Each program should include a comment section that includes, at a minimum, your name, the lab and exercise number, and a description of what the program accomplishes. - Submit a lab report (a Word document) containing the following information to the Dropbox for Week 1.
- Include your name and the exercise number.
- Specification: Include a brief description of what the program accomplishes, including its input, key processes, and output.
- Test Plan: Include a brief description of the method you used to confirm that your program worked properly. If necessary, include a clearly labeled table with test cases, predicted results, and actual results.
- Summary and Conclusions: Write a statement summarizing your predicted and actual output, and identify and explain any differences. For your conclusions, write at least one nontrivial paragraph that explains, in detail, either a significant problem you had and how you solved it or, if you had no significant problems, something you learned by doing the exercise.
- Answers to Lab Questions: Answer any and all of the lab questions included in the lab steps.
Each lab exercise should have a separate section in the lab-report document.
iLAB STEPS
STEP 1: Starting Visual Studio
Create a new Visual Studio empty project, and add one C++ source code file.
STEP 2: Coding
Enter the following source, which will set up the 2D array and the recommended variable declarations. It is up to the student to design and implement the remainder of the program code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 | // Programmer: (put your name here) // Course: COMP220 // Assignment: Two-Dimensional Arrays // Description: The program will use a 2D array and a random-number // generation to play Blackjack and keep track of a playing-card deck. // Input: User data entry and a playing-card deck represented as a two- // dimensional array // Output: A screen display showing the current card hands of each player // and the dealer, their score, win and lose status, and a final representation // of the card deck after the game is over # include <iostream> # include <iomanip> # include <windows.h> using namespace std; void main (void) { bool bPlayerDraw[5]; //Boolean to determine if player holds (F) //or draws card (T) char cPlay = 'N' ; //Character variable for play game input char cCardDeck[4][13]; //Character array representing the card deck int iCard; //0 = 2 card //1 = 3 card //2 = 4 card //3 = 5 card //4 = 6 card //5 = 7 card //6 = 8 card //7 = 9 card //8 = 10 card //9 = jack card //10 = queen card //11 = king card //12 = ace card int iNumberOfDraws = 0; //Number of rounds of card draws int iSuit; //Suit array index //0 = diamonds //1 = hearts //2 = clubs //3 = spades // ASCII character display reference for display card suit symbols //3 = heart symbol //4 = diamond symbol //5 = club symbol //6 = spade symbol int iNumberOfPlayers = 0; //Number of players in current game int iPlayerCount[5]; //Integer array to holder each player's count //iPlayer[0] is always the dealer int iHighestCount = 0; //Highest count for a single game int k, m; //integer loop counters srand(GetTickCount()); //Seed the random-number generator //Main game loop //Enter your code here... |
A sample output of the program is below:
Welcome to Honest Sam’s Blackjack Table Glad to have you back!
Enter the number of players in the game.
There must be at least one player but no more than four.
Number of players: 3
Card 1 | Card 2 | Card 3 | Total | Stats | |
---|---|---|---|---|---|
Dealer | J♦ | 10♦ | 9♣ | 29 | Lose |
Player 1 | K♣ | 2♠ | 5♥ | 17 | Lose |
Player 2 | J♣ | Q♥ | 10♥ | 30 | Lose |
Player 3 | 3♦ | 8♣ | K♦ | 21 | Win! |
STEP 3: Program Specifications
- There is always a dealer in the game. The dealer draws 3 cards.
- There must be at least one other player (you) and up to a maximum of four other players (all played by you).
- Each player will draw 3 cards.
- All the cards for each player, including the first card dealt, are displayed, along with the suit symbol: spades ♠, clubs ♣, hearts ♥, or diamonds ♦.
- Each game will start with a new, 52-card deck, which is modeled on a real deck of cards.
- The card deck has 52 cards with no jokers.
- The card deck is represented by a two-dimensional array of data-type character, where the first dimension represents the suit and the second dimension represents the card in the suit, such as the following.
char CardDeck[4][13]; - At the start of each game, each element of the two-dimensional array is initialized to a value of ” “, or the “space” character.
- The deck has four suits, represented by the following dimension indices.
- 0 = diamonds
- 1 = hearts
- 2 = clubs
- 3 = spades
- Each suit has 13 cards: 2, 3, 4, 5, 6, 7, 8, 9 ,10, jack, queen, king, and ace.
- Each card in a suit is represented by the following dimension indices.
- 0 = the 2 card
- 1 = the 3 card
- 2 = the 4 card
- 3 = the 5 card
- 4 = the 6 card
- 5 = the 7 card
- 6 = the 8 card
- 7 = the 9 card
- 8 = the 10 card
- 9 = the jack
- 10 = the queen
- 11 = the king
- 12 = the ace
- All the number cards are worth their face value (i.e., a 3 of diamonds is worth 3).
- All face cards are worth 10.
- An ace is worth either 1 or 11. Your final-score calculation must be able to handle this correctly for both the dealer and each player.
- A random-number generator must be used to select the suit and the card in the suit.
Once a card and suit are selected, the program should check if the value of that array element is a “space.”- If the array element = “space,” set the element equal to an integer, identifying the dealer or the player.
- 0 = dealer
- 1 = player 1
- 2 = player 2
- 3 = player 3
- 4 = player 4
- If the array element ! = “space,” then the random-number and card- checking process should repeat until a “card” or an array element is selected that is = “space.”
- If the array element = “space,” set the element equal to an integer, identifying the dealer or the player.
- Once a card is drawn during a game, it cannot be drawn again.
- If the user inputs to play the game, the next decision should be 1, 2, 3, or 4 players.
- The game ends when each player has 3 cards.
- The display should show each player’s (and the dealer’s) hand and update the display after each round of card draws.
- At the end of a game, the an updated balance.